NumberBox
It displays numbers in large boxes with title.
import mercury as mr
# displays large number in the box with border
mr.NumberBox(data=123, title="Large number")
# more boxes in one row
mr.NumberBox([
mr.NumberBox(data=123, title="First number"),
mr.NumberBox(data=456, title="Second number"),
mr.NumberBox(data=789, title="Third number")
])
The notebook with NumberBox
widgets:
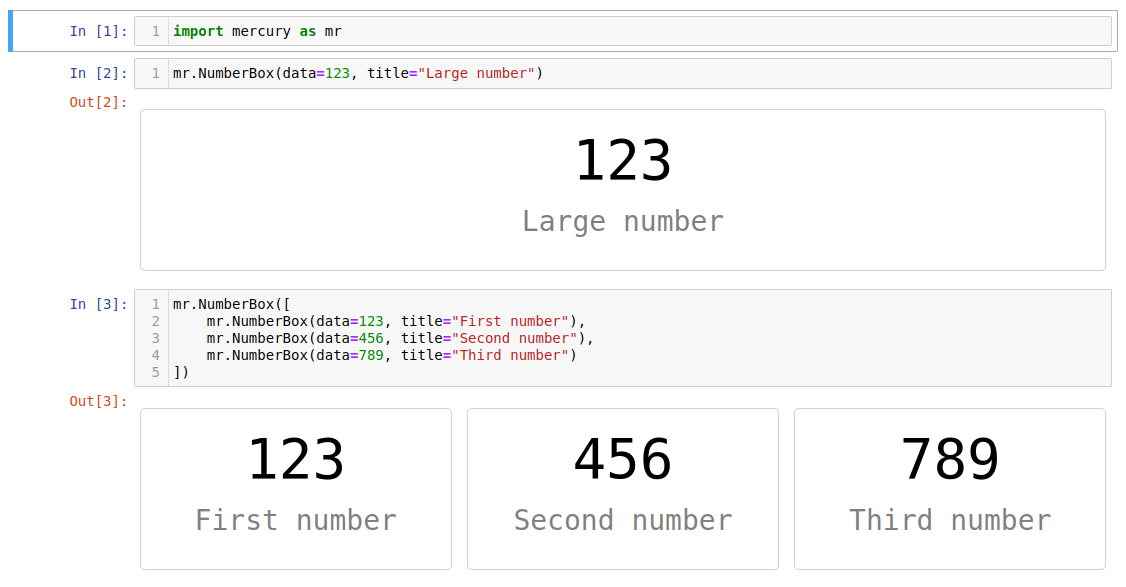
The Web App with NumberBox
widgets created from Python notebook:
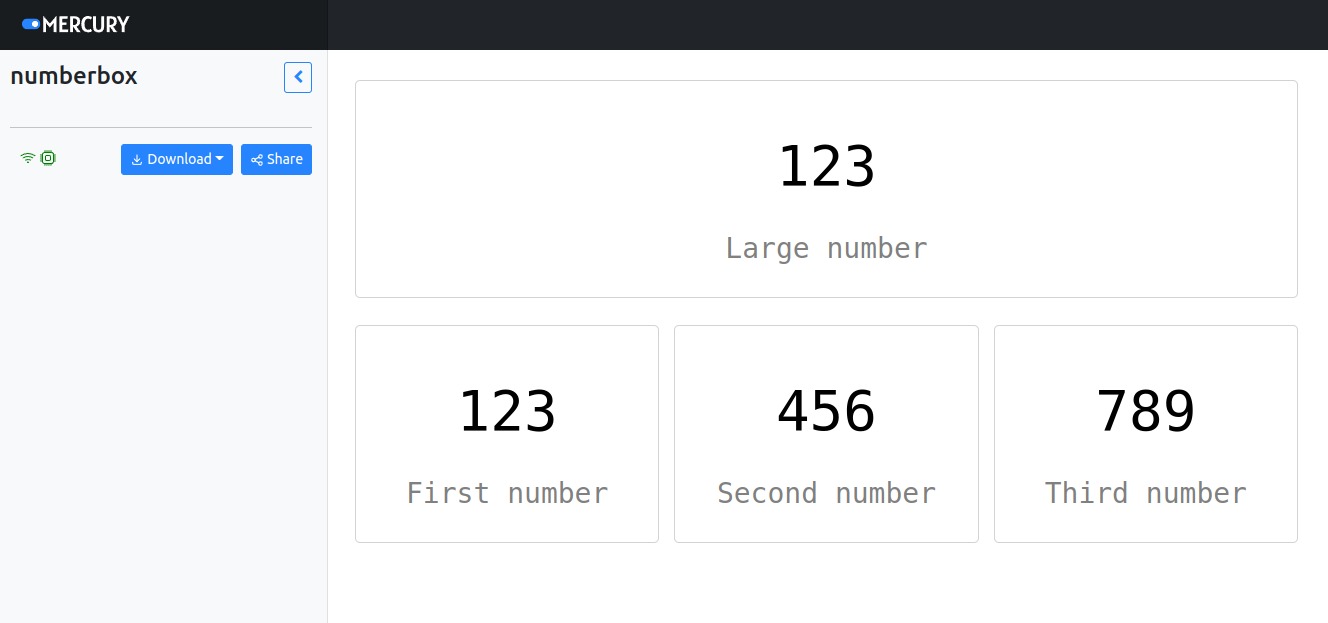
Parameters
- data (number or string or list) - data to be displayed (it has the largest font). It can be number or string. It also accepts list of
NumberBox
which will be displayed in the one row. - title (string) - description of the number in the box, it is displayed in the bottom.
- percent_change (number) - it is an optional argument that can show percent change. For positive numbers it is displayed with green color. For negative values it is displayed with red color.
- background_color (string) - it is an optional argument that sets the background color. Default is set to
white
. - border_color (string) - it is an optional argument that sets the border color. Default is set to
lightgray
. - data_color (string) - it is an optional argument that sets the color for data (large number). Default is set to
black
. - title_color (string) - it is an optional argument that sets colot for title (description). Default is set to
gray
.
Colors in the NumberBox
should be HTML color names (opens in a new tab) or hex strings (opens in a new tab). Examples:
black
,white
,green
,red
,#ff0000
,#00ff00
,#0000ff
.
Example App
Here is a simple app that uses NumberBox
and sets custom colors:
import mercury as mr
mr.NumberBox([
mr.NumberBox(data=123, title="First number", percent_change=100),
mr.NumberBox(data=456, title="Second number", percent_change=-50.1),
mr.NumberBox(data="🎉", title="Third number")
])
colors = ["#EA047E", "#FF6D28", "#FCE700", "#00F5FF"]
colors2 = ["#FFB84C", "#F266AB", "#A459D1", "#2CD3E1"]
colors3 = ["#2192FF", "#38E54D", "#9CFF2E", "#FDFF00"]
mr.NumberBox([
mr.NumberBox(data=123, title="First number", percent_change=100,
background_color=colors[0], border_color=colors[1],
data_color=colors[2], title_color=colors[3]),
mr.NumberBox(data=456, title="Second number", percent_change=-50.1,
background_color=colors2[0], border_color=colors2[1],
data_color=colors2[2], title_color=colors2[3]),
mr.NumberBox(data="🎉", title="Third number",
background_color=colors3[0], border_color=colors3[1],
data_color=colors3[2], title_color=colors3[3])
])
The view of the code in the Jupyter Notebook:
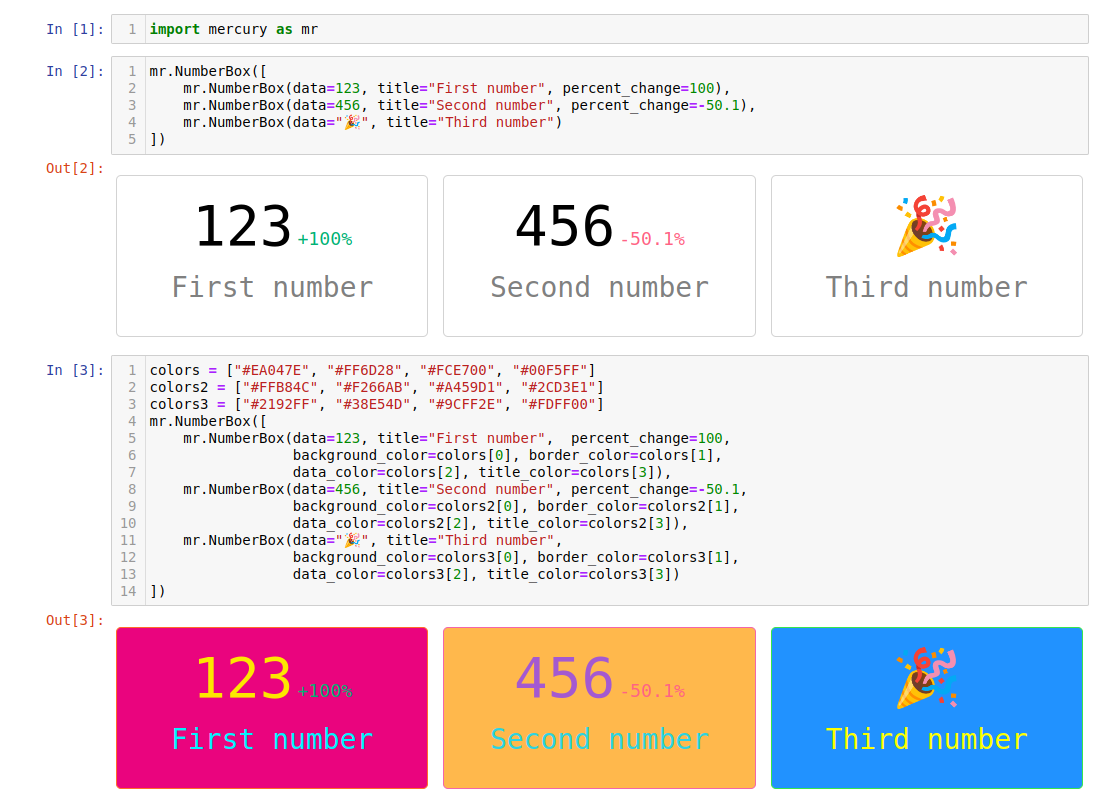
The view of the app in Mercury:
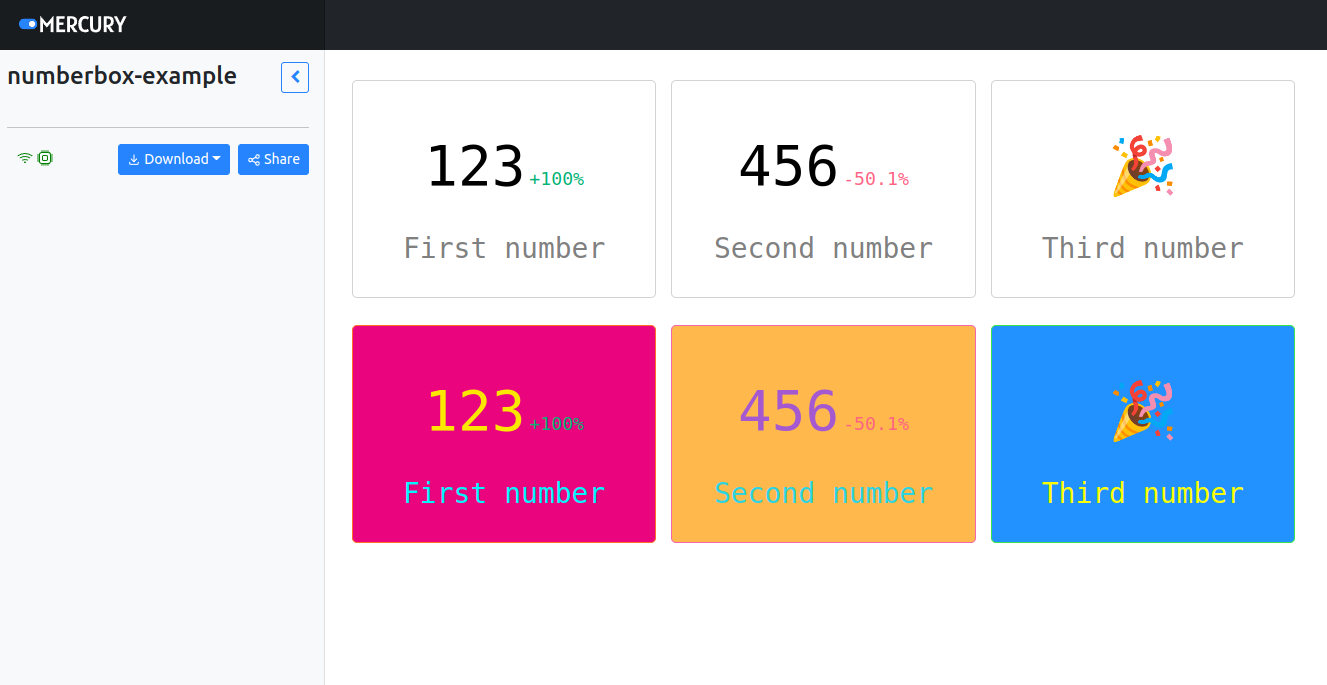
You can find the example code in our GitHub repository mljar/mercury-examples (opens in a new tab).