Select
Make your Notebook look better! You can add Select
widgets with option to select a single value, all of this with Select
(Mercury Widget).
Example code:
import mercury as mr
my_selection = mr.Select(value = "a", choices = ["a", "b", "c"], label = "Select option")
print(f"Selected value is: {my_selection.value}.")
Code explanation:
First step is importing Mercury. Then create variable (my_selection in our case) and asign the Select
widget to it. At the end print your results using my_selection.value
.
Parameters
- value (string) is a default selection. If value is not present in choices or if value is empty, it sets value to the first element of choices.
- choices (list of strings) is a place where you can set options which user chooses later. It can't be empty!
- label (string) is a title of selection widget.
- url_key (string) - set this value if you would like to set
Select
value with URL paramters. - disabled (boolean) - disable widget in the sidebar, default is set to
False
. - hidden (boolean) - hide widget in the sidebar, default is set to
False
.
Example of use
People often use Select
with if
construction:
First option selected:
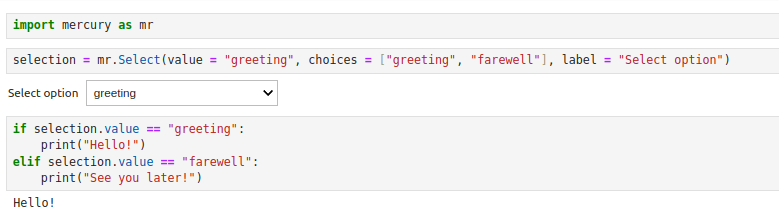
Second option selected:
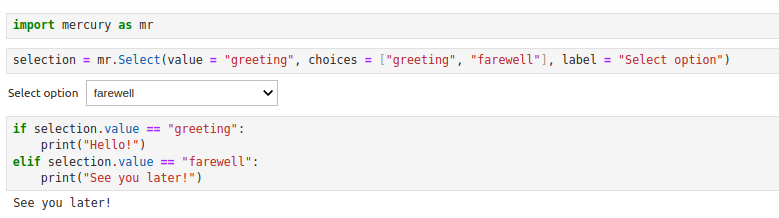
Code to copy:
import mercury as mr
selection = mr.Select(value = "greeting", choices = ["greeting", "farewell"], label = "Select option")
if selection.value == "greeting":
print("Hello!")
elif selection.value == "farewell":
print("See you later!")