Increment and decrement app in Jupyter Notebook and Mercury
We will create app with two buttons: Increment
and Decrement
. User will be able to control the value of the variable by clicking on buttons.
We will use Button
widget.
Required packages
You will need to install mercury
package to run this example.
Notebook
Let's import required packages:
import mercury as mr
Please define variable that will be updated on button click:
some_value = 0
We will add Increment
button:
increment = mr.Button(label="Increment", style="success")
There will be a Decrement
button:
decrement = mr.Button(label="Decrement", style="danger")
We will update the variable after buttons are clicked:
if increment.clicked:
some_value += 1
if decrement.clicked:
some_value -= 1
The last cell will display variable value:
print(f"Value is {some_value}")
The screenshot with code in the Jupyter Notebook:
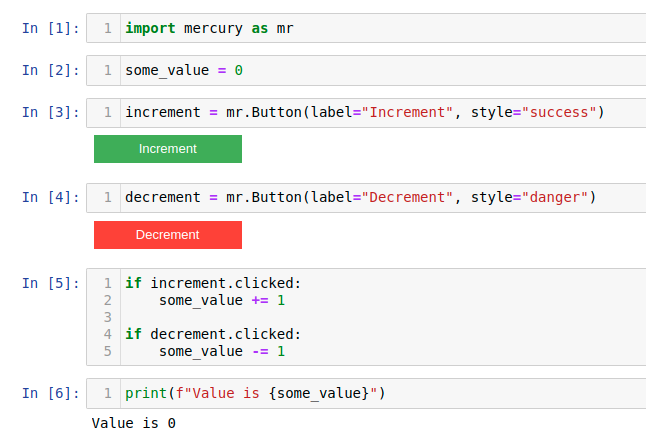
Please remember, that widget update doesn't trigger automatic cell re-execution during notebook development in Jupyter Notebook.
Cells are automatically re-executed in Mercury.
Mercury App
Please start Mercury in the same directory as notebook:
mercury run
The Mercury Site is available at http://127.0.0.1:8000
. Please open notebook with dynamic widgets, you can play with widgets and see the updated output:
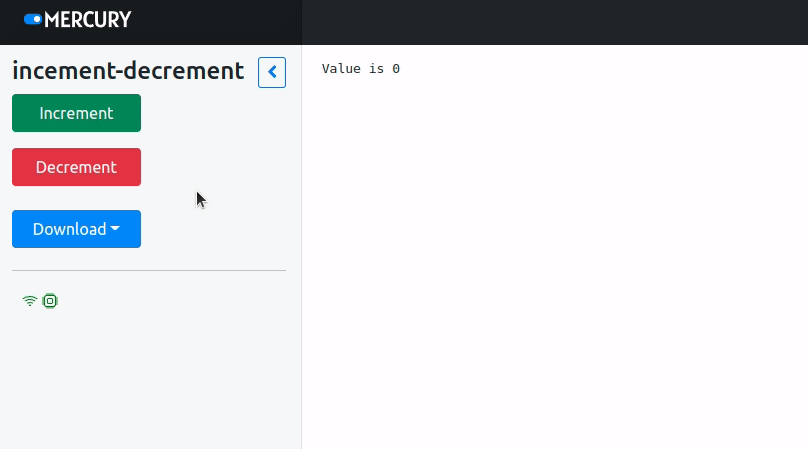
Button click triggers automatic cells re-execution. Cell with widget definition and below are re-executed. If button was clicked the .clicked
attribute will be True
.
This attribute will be True
only once! After we read it, it will be set back to False
.
The Download
button is to export executed result as PDF or HTML file.
The notebook code is available in mercury-examples (opens in a new tab) GitHub repository.