Matplotlib Histogram Chart
Introduction
Matplotlib
is a library for making charts, which works very well with Jupyter Notebook. One of them is called a Histogram Chart. We've got a few examples ready to show you, so you can see what they look like and how they work:
- simple histogram chart
- histogram chart with
numpy
data - histogram chart with
pandas
data - an interactive histogram chart
If you need any information about Matplotlib check their docs: Matplotlib Docs (opens in a new tab).
All of code examples are availabe as Jupyter Notebooks in our GitHub repositiory:
- Matplotlib Histogram Chart (opens in a new tab)
- Matplotlib Interactive Histogram Chart (opens in a new tab)
Histogram Chart
Example with using only matplotlib
package:
# import packages
import matplotlib.pyplot as plt
import random
# create data
x = [random.gauss(0,1) for _ in range(1000)]
# plot
plt.hist(x)
plt.show()
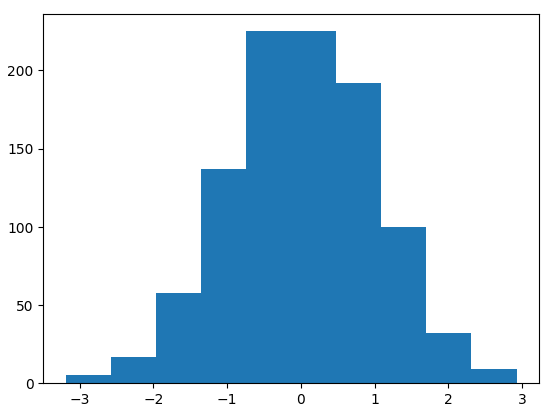
Histogram Chart with Numpy Data
You can turn numpy
data into histogram chart thanks to matplotlib
library:
# import packages
import matplotlib.pyplot as plt
import numpy as np
# create data
x = np.random.randn(100)
# plot
plt.hist(x)
plt.show()
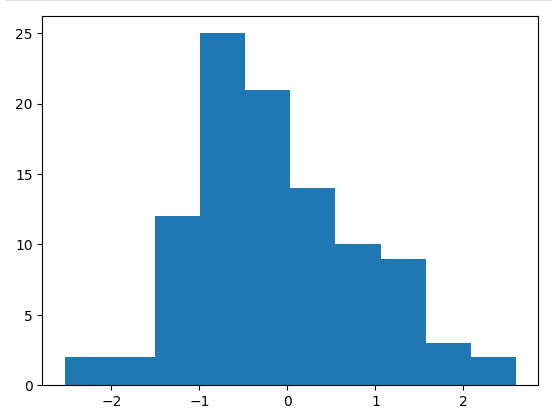
Histogram Chart with Pandas Data
Display pandas
data as a histogram chart created with matplotlib
:
# import packages
import matplotlib.pyplot as plt
import pandas as pd
import random
# create data
df = pd.DataFrame({'numbers': [random.gauss(0,1) for _ in range(1000)]})
# plot
plt.hist(df['numbers'])
plt.show()
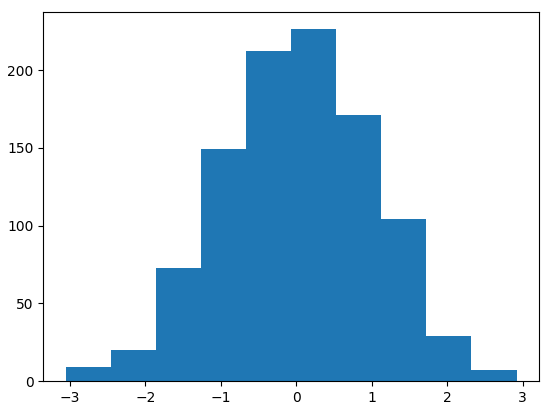
Interactive Histogram Chart
Static charts are boring, what about creating an interactive histogram chart? It's possible with matplotlib
and mercury
packages. You can choose what color do you want the chart to be using mercury
widgets. In this example we used Select (opens in a new tab):
# import packages
import matplotlib.pyplot as plt
import mercury as mr
import random
# mercury widget
selection = mr.Select(value="blue", choices=["blue","green","red"])
# create data
x = [random.gauss(0,1) for _ in range(1000)]
# plot
plt.hist(x, color=selection.value)
plt.show()
Now, you can turn your Jupyter Notebook into Web App without additional code changes! Here is a video which presents how it will look:
Deploying Web App is very easy that you can do it in 3 steps:
Login to Mercury Cloud
If you don't have account, you can create it here: Mercury Cloud (opens in a new tab).
Create new site
Create new or use an existing site.
Upload your notebook
Upload the notebook with code.
Congrats! You just created your own Web App and you can share your Jupyter Notebooks with nontechnical users. If you need more information about deploying the Web App check Mercury Cloud Documentation (opens in a new tab).