Seaborn Scatter Chart
Introduction
Seaborn
is a tool used for creating visual charts, specifically designed to work smoothly with Jupyter Notebook. Among its offerings is the Scatter Chart, a commonly used visualization method. We've prepared some examples to illustrate how they appear and function for your reference.
- simple scatter chart
- scatter chart with
numpy
data - scatter chart with
pandas
data - an interactive scatter chart
If you need any information about Seaborn check their docs: Seaborn Docs (opens in a new tab).
All of code examples are availabe as Jupyter Notebooks in our GitHub repositiory:
Scatter Chart
Example with just seaborn
:
# import packages
import seaborn as sns
# create data
x = [3, 9, 9, 7, 2, 10, 9, 6, 6, 10]
# plot
_ = sns.scatterplot(x)
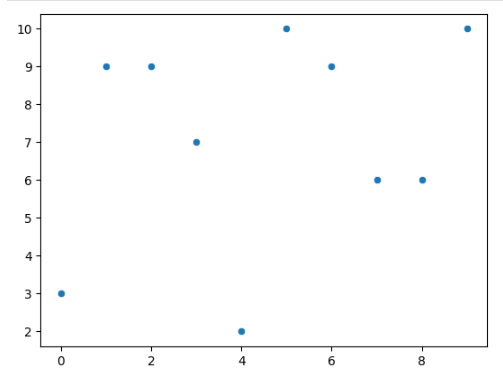
Scatter Chart with Numpy Data
Show numpy
data as scatter chart using seaborn
:
# import packages
import seaborn as sns
import numpy as np
# create data
x = np.array([3, 9, 7, 4, 3, 1, 10, 7, 10, 6])
# plot
_ = sns.scatterplot(x)
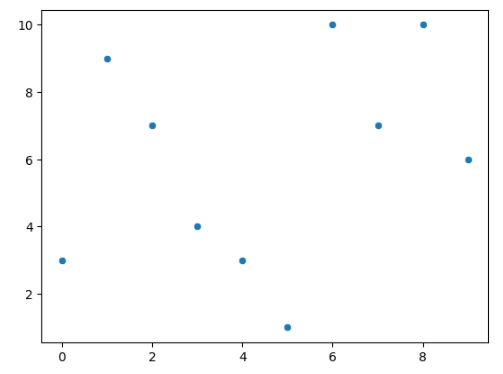
Scatter Chart with Pandas Data
Create a scatter chart using seaborn
with pandas
data:
# import packages
import seaborn as sns
import pandas as pd
# create data
df = pd.DataFrame({ 'value': [4, 3, 6, 4, 1, 5, 10, 2, 1, 3]})
# plot
_ = sns.scatterplot(df)
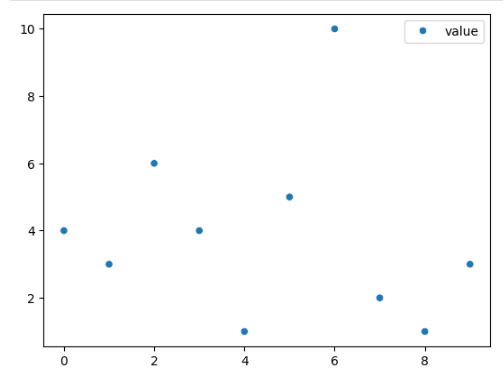
Interactive Scatter Chart
Static charts are boring. Let's create an interactive scatter chart using seaborn
and mercury
packages. You can modify data range conveniently with mercury widgets. In this example we used Slider (opens in a new tab).
# import packages
import seaborn as sns
import mercury as mr
# mercury widget
slider = mr.Slider(value=10, min=8, max=20, label="How many dots?")
# create data
all_x = [22, 6, 17, 13, 10, 3, 17, 21, 22, 22, 9, 11, 10, 12, 16, 16, 4, 6, 14, 9]
x = all_x[0:slider.value]
# plot
_ = sns.scatterplot(x)
Now, you can transform your Jupyter Notebook into a Web App effortlessly! Watch this video to see how it look.
Deploying Web App is very easy that you can do it in 3 steps:
Login to Mercury Cloud
If you don't have account, you can create it here: Mercury Cloud (opens in a new tab).
Create new site
Create new or use an existing site.
Upload your notebook
Upload the notebook with code.
Congrats! You just created your own Web App and you can share your Jupyter Notebooks with nontechnical users. If you need more information about deploying the Web App check Mercury Cloud Documentation (opens in a new tab).