Matplotlib Hexbin Chart
Introduction
Matplotlib
is a library for making charts, which works very well with Jupyter Notebook. One of them is called a Hexbin Chart. We've got a few examples ready to show you, so you can see what they look like and how they work.
- hexbin chart with
numpy
data - hexbin chart with
pandas
data - an interactive hexbin chart
If you need any information about Matplotlib check their docs: Matplotlib Docs (opens in a new tab).
All of code examples are availabe as Jupyter Notebooks in our GitHub repositiory:
- Matplotlib Hexbin Chart (opens in a new tab)
- Matplotlib Interactive Hexbin Chart (opens in a new tab)
Hexbin Chart with Numpy Data
Display numpy
data as hexbin chart using matplotlib
library:
# import packages
import numpy as np
import matplotlib.pyplot as plt
# create data
np.random.seed()
x = np.random.standard_normal(100)
y = np.random.standard_normal(100)
# plot
plt.hexbin(x, y, gridsize=(5, 3))
plt.show()
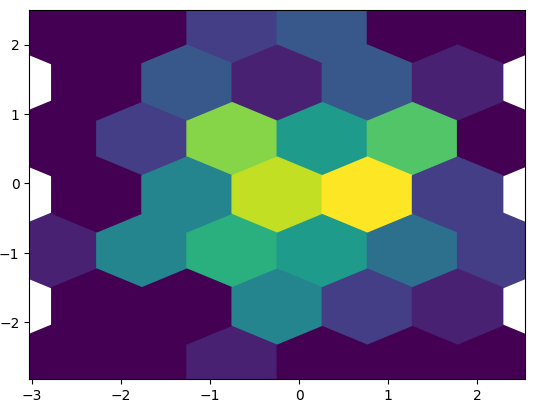
Hexbin Chart with Pandas Data
Turn pandas
data into hexbin chart created with matplotlib
:
# import packages
import pandas as pd
import random
import matplotlib.pyplot as plt
# create data
df = pd.DataFrame({'x': pd.Series(range(100)).apply(lambda x: random.gauss(0, 1)),
'y': pd.Series(range(100)).apply(lambda y: random.gauss(0, 1))})
# plot
plt.hexbin(df['x'],df['y'], gridsize=(5, 3))
plt.show()
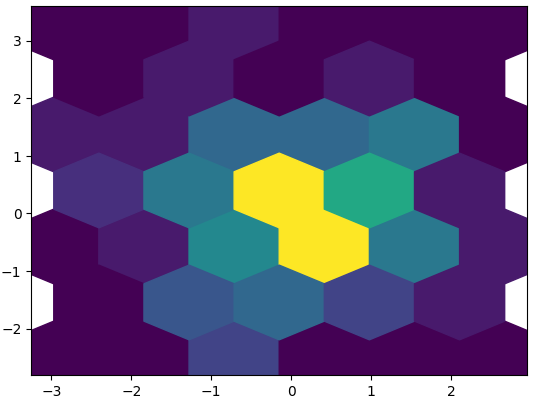
Interactive Hexbin Chart
Static charts are boring, what about creating an interactive hexbin chart? It's possible with matplotlib
and mercury
packages. You can modify gridsize with mercury
widgets. In this example we used Slider (opens in a new tab):
# import packages
import mercury as mr
import matplotlib.pyplot as plt
import numpy as np
# mercury widget
slider = mr.Slider(value=4, min=3, max=8, label="Set gridsize:")
# create data
np.random.seed()
x = np.random.standard_normal(200)
y = np.random.standard_normal(200)
# plot
plt.hexbin(x, y, gridsize=(slider.value, slider.value))
plt.show()
Now, you can turn your Jupyter Notebook into Web App without additional code changes! Here is a video which presents how it will look:
Deploying Web App is very easy that you can do it in 3 steps:
Login to Mercury Cloud
If you don't have account, you can create it here: Mercury Cloud (opens in a new tab).
Create new site
Create new or use an existing site.
Upload your notebook
Upload the notebook with code.
Congrats! You just created your own Web App and you can share your Jupyter Notebooks with nontechnical users. If you need more information about deploying the Web App check Mercury Cloud Documentation (opens in a new tab).